设计模式之组合模式(Composite)摘录
Composite:
(1)、意图:将对象组合成树形结构以表示“部分--整体”的层次结构。Composite使得用户对单个对象和组合对象的使用具有一致性。
(2)、适用性:A、你想表示对象的部分--整体层次结构;B、你希望用户忽略组合对象与单个对象的不同,用户将统一地使用组合结构中的所有对象。
(3)、优缺点:
A、定义了包含基本对象和组合对象的类层次结构:基本对象可以被组合成更复杂的组合对象,而这个组合对象又可以被组合,这样不断的递归下去。客户代码中,任何用到基本对象的地方都可以使用组合对象。
B、简化客户代码:客户可以一致地使用组合结构和单个对象。通常用户不知道(也不关心)处理的是一个叶节点还是一个组合组件。这就简化了客户代码,因为在定义组合的那些类中不需要写一些充斥着选择语句的函数。
C、使得更容易增加新类型的组件:新定义的Composite或Leaf子类自动地与已有的结构和客户代码一起工作,客户程序不需要因新的Component类而改变。
D、使你的设计变得更加一般化:有时你希望一个组合只能有某些特定的组件。使用Composite时,你不能依赖类型系统施加这些约束,而必须在运行时刻进行检查。
(4)、注意事项:
A、显示的父部件引用:保持从子部件到父部件的引用能简化组合结构的遍历和管理。父部件引用可以简化结构的上移和组件的删除,同时父部件引用也支持Chain
of Responsibility。
B、共享组件:共享组件是很有用的,比如它可以减少对存贮的需求。但是当一个组件只有一个父部件时,很难共享组件。
C、最大化Component接口:Composite模式的目的之一是使得用户不知道他们正在使用的具体的Leaf和Composite类。为了达到这一目的,Composite类应为Leaf和Composite类尽可能多定义一些公共操作。Composite类通常为这些操作提供缺省的实现,而Leaf和Composite子类可以对它们进行重定义。
D、声明管理子部件的操作。
E、Component是否应该实现一个Component列表:你可能希望在Component类中将子节点集合定义为一个实例变量,而这个Component类中也声明了一些操作对子节点进行访问和管理。但是在基类中存放子类指针,对叶节点来说会导致空间浪费,因为叶节点根本没有子节点。只有当该结构中子类数目相对较少时,才值得使用这种方法。
F、子部件排序:如果需要考虑子节点的顺序时,必须仔细地设计对子节点的访问和管理接口,以便管理子节点序列。Iterator模式可以在这方面给予一些指导。
G、使用高速缓冲存贮改善性能:如果你需要对组合进行频繁的遍历或查找,Composite类可以缓冲存储对它的子节点进行遍历或查找相关信息。H、应该由谁删除Component:在没有垃圾回收机制的语言中,当一个Composite被销毁时,通常最好由Composite负责删除其子节点。但有一种情况除外,即Leaf对象不会改变,因此可以被共享。
I、存贮组件最好用哪一种数据结构:Composite可使用多种数据结构存贮它们的子节点,包括连接列表、树、数组和hash表。数据结构的选择取决于效率。事实上,使用通用数据结构根本没有必要。有时对每个子节点,Composite都有一个变量与之对应,这就要求Composite的每个子类都要实现自己的管理接口。
(5)、相关模式:
A、通常部件----父部件连接用于Responsibilityof Chain模式。
B、Decorator模式经常与Composite模式一起使用。当装饰和组合一起使用时,它们通常有一个公共的父类。因此装饰必须支持具有Add、Remove和GetChild操作的Component接口。
C、Flyweight让你共享组件,但不再能引用他们的父部件。
D、Iterator可用来遍历Composite.
E、Visitor将本来应该分布在Composite和Leaf类中的操作和行为局部化。
Composite模式在实现中有一个问题就是要提供对于子节点(Leaf)的管理策略,可以提供的实现方式有:vector、数组、链表、Hash表等。
Composite模式通过和Decorator模式有着类似的结构图,但是Composite模式旨在构造类,而Decorator模式重在不生成子类即可给对象添加职责。Decorator模式重在修饰,而Composite模式重在表示。
示例代码1:
#include <iostream> #include <string> #include <vector> using namespace std; class Component { public: string m_strName; Component(string strName) { m_strName = strName; } virtual void Add(Component* com) = 0; virtual void Display(int nDepth) = 0; }; class Leaf : public Component { public: Leaf(string strName) : Component(strName) {} virtual void Add(Component* com) { cout<<"leaf can't add"<<endl; } virtual void Display(int nDepth) { string strtemp; for (int i = 0; i < nDepth; i ++) strtemp += "-"; strtemp += m_strName; cout<<strtemp<<endl; } }; class Composite : public Component { private: vector<Component*> m_component; public: Composite(string strName) : Component(strName) {} virtual void Add(Component* com) { m_component.push_back(com); } virtual void Display(int nDepth) { string strtemp; for (int i = 0; i < nDepth; i ++) strtemp += "-"; strtemp += m_strName; cout<<strtemp<<endl; vector<Component*>::iterator p = m_component.begin(); while (p != m_component.end()) { (*p)->Display(nDepth + 2); p ++; } } }; //客户端 int main() { Composite* p = new Composite("小王"); p->Add(new Leaf("小李")); p->Add(new Leaf("小赵")); Composite* p1 = new Composite("小小五"); p1->Add(new Leaf("大三")); p->Add(p1); p->Display(1); /*result -小王 ---小李 ---小赵 ---小小五 -----大三 */ return 0; } |
示例代码2:
#include <iostream> #include <string> #include <vector> using namespace std; class Company { protected: string m_strName; public: Company(string strName) { m_strName = strName; } virtual void Add(Company* c) = 0; virtual void Display(int nDepth) = 0; virtual void LineOfDuty() = 0; }; class ConcreteCompany : public Company { private: vector<Company*> m_company; public: ConcreteCompany(string strName) : Company(strName) {} virtual void Add(Company* c) { m_company.push_back(c); } virtual void Display(int nDepth) { string strtemp; for (int i = 0; i < nDepth; i ++) strtemp += "-"; strtemp += m_strName; cout<<strtemp<<endl; vector<Company*>::iterator p = m_company.begin(); while (p != m_company.end()) { (*p)->Display(nDepth + 1); p ++; } } virtual void LineOfDuty() { vector<Company*>::iterator p = m_company.begin(); while (p != m_company.end()) { (*p)->LineOfDuty(); p ++; } } }; class HrDepartment : public Company { public: HrDepartment(string strname) : Company(strname) {} virtual void Display(int nDepth) { string strtemp; for (int i = 0; i < nDepth; i ++) strtemp += "-"; strtemp += m_strName; cout<<strtemp<<endl; } virtual void Add(Company* c) { cout<<"error"<<endl; } virtual void LineOfDuty() { cout<<m_strName<<":招聘人才"<<endl; } }; //客户端 int main() { ConcreteCompany* p = new ConcreteCompany("清华大学"); p->Add(new HrDepartment("清华大学人才部")); ConcreteCompany* p1 = new ConcreteCompany("数学系");; p1->Add(new HrDepartment("数学系人才部")); ConcreteCompany* p2 = new ConcreteCompany("物理系"); p2->Add(new HrDepartment("物理系人才部")); p->Add(p1); p->Add(p2); p->Display(1); p->LineOfDuty(); /*result -清华大学 --清华大学人才部 --数学系 ---数学系人才部 --物理系 ---物理系人才部 清华大学人才部:招聘人才 数学系人才部:招聘人才 物理系人才部:招聘人才 */ return 0; } |
示例代码3:
Component.h:
#ifndef _COMPONENT_H_ #define _COMPONENT_H_ class Component { public: Component(); virtual ~Component(); public: virtual void Operation() = 0; virtual void Add(const Component&); virtual void Remove(const Component&); virtual Component* GetChild(int); protected: private: }; #endif//~_COMPONENT_H_ |
Component.cpp:
#include "Component.h" Component::Component() { } Component::~Component() { } void Component::Add(const Component&) { } Component* Component::GetChild(int index) { return 0; } void Component::Remove(const Component& com) { }
|
Composite.h:
#ifndef _COMPOSITE_H_ #define _COMPOSITE_H_ #include "Component.h" #include <vector> using namespace std; class Composite : public Component { public: Composite(); ~Composite(); public: void Operation(); void Add(Component* com); void Remove(Component* com); Component* GetChild(int index); protected: private: vector<Component*> comVec; }; #endif//~_COMPOSITE_H_ |
Composite.cpp:
#include "Composite.h" #include "Component.h" #define NULL 0 //define NULL POINTOR Composite::Composite() { //vector<Component*>::iterator itend = comVec.begin(); } Composite::~Composite() { } void Composite::Operation() { vector<Component*>::iterator comIter = comVec.begin(); for (; comIter != comVec.end(); comIter ++) (*comIter)->Operation(); } void Composite::Add(Component* com) { comVec.push_back(com); } void Composite::Remove(Component* com) { //comVec.erase(&com); } Component* Composite::GetChild(int index) { return comVec[index]; } |
Leaf.h:
#include "Composite.h" #include "Component.h" #define NULL 0 //define NULL POINTOR Composite::Composite() { //vector<Component*>::iterator itend = comVec.begin(); } Composite::~Composite() { } void Composite::Operation() { vector<Component*>::iterator comIter = comVec.begin(); for (; comIter != comVec.end(); comIter ++) (*comIter)->Operation(); } void Composite::Add(Component* com) { comVec.push_back(com); } void Composite::Remove(Component* com) { //comVec.erase(&com); } Component* Composite::GetChild(int index) { return comVec[index]; } |
Leaf.cpp:
#include "Leaf.h" #include <iostream> using namespace std; Leaf::Leaf() { } Leaf::~Leaf() { } void Leaf::Operation() { cout<<"Leaf Operation ..."<<endl; } |
main.cpp:
#include "Component.h" #include "Composite.h" #include "Leaf.h" #include <iostream> using namespace std; int main() { Leaf* l = new Leaf(); l->Operation(); Composite* com = new Composite(); com->Add(l); com->Operation(); Component* ll = com->GetChild(0); ll->Operation(); /*result Leaf Operation ... Leaf Operation ... Leaf Operation ... */ return 0; } |
组合模式结构图:
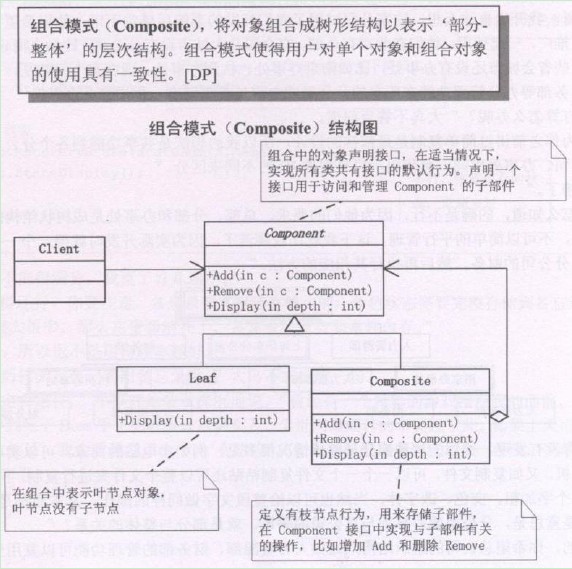
设计模式之享元模式(Flyweight)摘录
Flyweight:(1)、意图: 运用共享技术有效地支持大量细粒度的对象。
(2)、Flyweight是一个共享对象,它可以同时在多个场景(context)中使用,并且在每个场景中flyweight都可以作为一个独立的对象----这一点与非共享对象的实例没有区别。Flyweight模式对那些通常因为数量太大而难以用对象来表示的概念或实体进行建模。
(3)、适用性:当一下情况都成立时使用Flyweight模式:A、一个应用程序使用了大量对象;B、完全由于使用大量的对象,造成很大的存储开销;C、对象的大多数状态都可变为外部状态;D、如果删除对象的外部状态,那么可以用相对较少的共享对象取代很大组对象;E、应用程序不依赖于对象标识。由于Flyweight对象可以被共享,对于概念上明显有别的对象,标识测试将返回真值。
(4)、注意事项:A、删除外部状态:该模式的可用性在很大程度上取决于是否容易识别外部状态并将它从共享对象中删除。B、管理共享对象:因为对象是共享的,用户不能直接对它进行实例化,因此Flyweight-Factory可以帮助用户查找某个特定的Flyweight对象。
(5)、相关模式:Flyweight模式通常和Composite模式结合起来,用共享叶节点的有向无环图实现一个逻辑上的层次结构。通常,最好用Flyweight实现State和Strategy对象。
运用共享技术有效地支持大量细粒度的对象(对于C++来说就是共用一个内存块,对象指针指向同一个地方)。如果一个应用程序使用了大量的对象,而这些对象造成了很大的存储开销就应该考虑使用。还有就是对象的大多数状态可以外部状态,如果删除对象的外部状态,那么可以用较少的共享对象取代多组对象,此时可以考虑使用享元。
Flyweight模式在实现过程中主要是要为共享对象提供一个存放的”仓库”(对象池)。
示例代码1:
#include <string> #include <vector> #include <iostream> using namespace std; //抽象的网站 class WebSite { public: virtual void Use() = 0; }; //具体的共享网站 class ConcreteWebSite : public WebSite { private: string name; public: ConcreteWebSite(string strName) { name = strName; } virtual void Use() { cout<<"网站分类:"<<name<<endl; } }; //不共享的网站 class UnShareWebSite : public WebSite { private: string name; public: UnShareWebSite(string strName) { name = strName; } virtual void Use() { cout<<"不共享的网站:"<<name<<endl; } }; //网站工厂类,用于存放共享的WebSite对象 class WebFactory { private: vector<WebSite*> websites; public: WebSite* GetWeb() { vector<WebSite*>::iterator p = websites.begin(); return *p; } WebFactory() { websites.push_back(new ConcreteWebSite("测试")); } }; //客户端 int main() { WebFactory* f = new WebFactory(); WebSite* ws = f->GetWeb(); ws->Use(); WebSite* ws2 = f->GetWeb(); ws2->Use(); /*result 网站分类:测试 网站分类:测试 */ return 0; } |
示例代码2:
Flyweight.h:
#ifndef _FLYWEIGHT_H_ #define _FLYWEIGHT_H_ #include <string> using namespace std; class Flyweight { public: virtual ~Flyweight(); virtual void Operation(const string& extrinsicState); string GetIntrinsicState(); protected: Flyweight(string intrinsicState); private: string _intrinsicState; }; class ConcreteFlyweight : public Flyweight { public: ConcreteFlyweight(string intrinsicState); ~ConcreteFlyweight(); void Operation(const string& extrinsicState); protected: private: }; #endif//~_FLYWEIGHT_H_ |
Flyweight.cpp:
#include "Flyweight.h" #include <iostream> using namespace std; Flyweight::Flyweight(string intrinsicState) { this->_intrinsicState = intrinsicState; } Flyweight::~Flyweight() { } void Flyweight::Operation(const string& extrinsicState) { } string Flyweight::GetIntrinsicState() { return this->_intrinsicState; } ConcreteFlyweight::ConcreteFlyweight(string intrinsicState) : Flyweight(intrinsicState) { cout<<"ConcreteFlyweight Build ..."<<intrinsicState<<endl; } ConcreteFlyweight::~ConcreteFlyweight() { } void ConcreteFlyweight::Operation(const string& extrinsicState) { cout<<"ConcreteFlyweight: ["<<this->GetIntrinsicState()<<"] ["<<extrinsicState<<"]"<<endl; } |
FlyweightFactory.h:
#ifndef _FLYWEIGHTFACTORY_H_ #define _FLYWEIGHTFACTORY_H_ #include "Flyweight.h" #include <string> #include <vector> using namespace std; class FlyweightFactory { public: FlyweightFactory(); ~FlyweightFactory(); Flyweight* GetFlyweight(const string& key); protected: private: vector<Flyweight*> _fly; }; #endif//~_FLYWEIGHTFACTORY_H_ |
FlyweightFactory.cpp:
#include "FlyweightFactory.h" #include <iostream> #include <string> #include <cassert> using namespace std; FlyweightFactory::FlyweightFactory() { } FlyweightFactory::~FlyweightFactory() { } Flyweight* FlyweightFactory::GetFlyweight(const string& key) { vector<Flyweight*>::iterator it = _fly.begin(); for (; it != _fly.end(); it ++) { if ((*it)->GetIntrinsicState() == key) { cout<<"already created by users ..."<<endl; return *it; } } Flyweight* fn = new ConcreteFlyweight(key); _fly.push_back(fn); return fn; } |
main.cpp:
#include "Flyweight.h" #include "FlyweightFactory.h" #include <iostream> using namespace std; int main() { FlyweightFactory* fc = new FlyweightFactory(); Flyweight* fw1 = fc->GetFlyweight("hello"); Flyweight* fw2 = fc->GetFlyweight("world!"); Flyweight* fw3 = fc->GetFlyweight("hello"); /*result ConcreteFlyweight Build ...hello ConcreteFlyweihgt Build ...world! already created by users ... */ return 0; }
|
享元模式结构图:
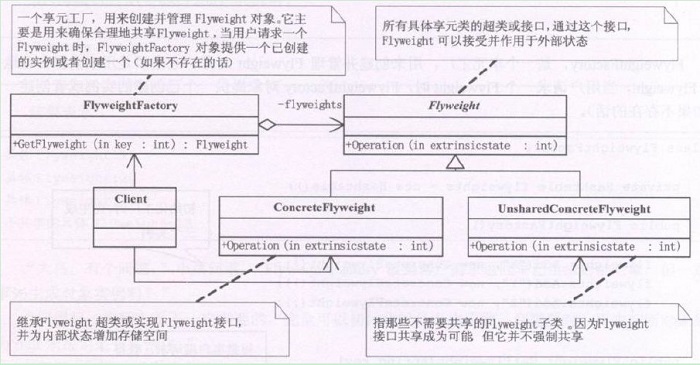
|