动画效果提供了状态或页面转换时流畅的用户体验,在iOS系统中,咱们不需要自己编写绘制动画的代码,Core
Animation提供了丰富的api来实现你需要的动画效果。
UIKit只用UIView来展示动画,动画支持UIView下面的这些属性改变:
- frame
- bounds
- center
- transform
- alpha
- backgroundColor
- contentStretch
1、commitAnimations方式使用UIView动画
- (void)viewDidLoad
{
[super viewDidLoad];
UIButton *button = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button setTitle:@"改变" forState:UIControlStateNormal];
button.frame = CGRectMake(10, 10, 60, 40);
[button addTarget:self action:@selector(changeUIView) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button];
}
- (void)changeUIView{
[UIView beginAnimations:@"animation" context:nil];
[UIView setAnimationDuration:1.0f];
[UIView setAnimationCurve:UIViewAnimationCurveEaseInOut];
[UIView setAnimationTransition:UIViewAnimationTransitionFlipFromRight forView:self.view cache:YES];
[UIView commitAnimations];
} |
下面是点击改变后的效果(两种):
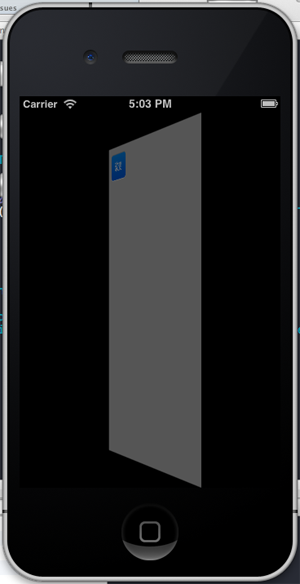 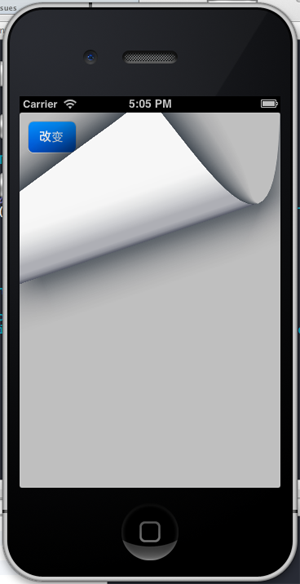
动画的常量有一下四种
UIViewAnimationTransitionNone,
UIViewAnimationTransitionFlipFromLeft,
UIViewAnimationTransitionFlipFromRight,
UIViewAnimationTransitionCurlUp,
UIViewAnimationTransitionCurlDown, |
1.2 交换本视图控制器中2个view位置
[self.view exchangeSubviewAtIndex:1
withSubviewAtIndex:0];
先添加两个view ,一个redview 一个yellowview
- (void)viewDidLoad
{
[super viewDidLoad];
UIView *redView = [[UIView alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
redView.backgroundColor = [UIColor redColor];
[self.view addSubview:redView];
UIView *yellowView = [[UIView alloc] initWithFrame:[[UIScreen mainScreen] bounds]];
yellowView.backgroundColor = [UIColor yellowColor];
[self.view addSubview:yellowView];
UIButton *button = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button setTitle:@"改变" forState:UIControlStateNormal];
button.frame = CGRectMake(10, 10, 300, 40);
[button addTarget:self action:@selector(changeUIView) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button];
UIButton *button1 = [UIButton buttonWithType:UIButtonTypeRoundedRect];
[button1 setTitle:@"改变1" forState:UIControlStateNormal];
button1.frame = CGRectMake(10, 60, 300, 40);
[button1 addTarget:self action:@selector(changeUIView1) forControlEvents:UIControlEventTouchUpInside];
[self.view addSubview:button1];
} |
- (void)changeUIView1{
[UIView beginAnimations:@"animation" context:nil];
[UIView setAnimationDuration:1.0f];
[UIView setAnimationCurve:UIViewAnimationCurveEaseInOut];
[UIView setAnimationTransition:UIViewAnimationTransitionCurlDown forView:self.view cache:YES];
// 交换本视图控制器中2个view位置
[self.view exchangeSubviewAtIndex:1 withSubviewAtIndex:0];
[UIView commitAnimations];
} |
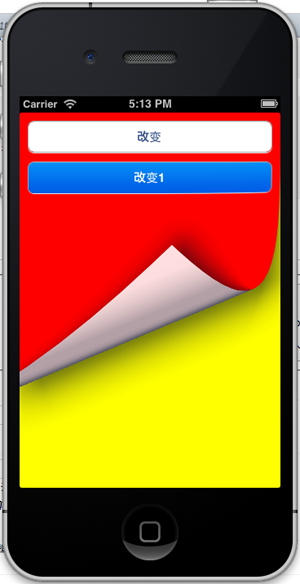
这样看起来就像两页一样了。
1.3 、 [UIView setAnimationDidStopSelector:@selector(animationFinish:)];
在commitAnimations消息之前,可以设置动画完成后的回调,设置方法是:
[UIView setAnimationDidStopSelector:@selector(animationFinish:)];
2、使用:CATransition
- (void)changeUIView2{
CATransition *transition = [CATransition animation];
transition.duration = 2.0f;
transition.type = kCATransitionPush;
transition.subtype = kCATransitionFromTop;
[self.view exchangeSubviewAtIndex:1 withSubviewAtIndex:0];
[self.view.layer addAnimation:transition forKey:@"animation"];
} |
transition.type 的类型可以有
淡化、推挤、揭开、覆盖
NSString * const kCATransitionFade;
NSString * const kCATransitionMoveIn;
NSString * const kCATransitionPush;
NSString * const kCATransitionReveal;
这四种,
transition.subtype
也有四种
NSString * const kCATransitionFromRight;
NSString * const kCATransitionFromLeft;
NSString * const kCATransitionFromTop;
NSString * const kCATransitionFromBottom;
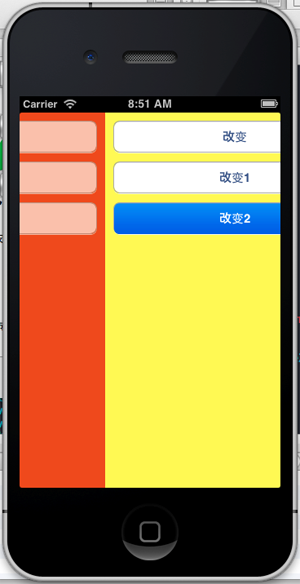 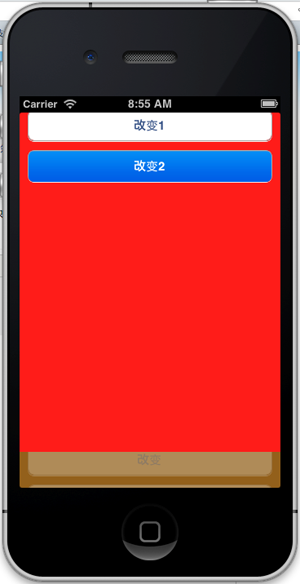
2.2 私有的类型的动画类型:
立方体、吸收、翻转、波纹、翻页、反翻页、镜头开、镜头关
animation.type = @"cube"
animation.type = @"suckEffect";
animation.type = @"oglFlip";//不管subType is "fromLeft" or "fromRight",official只有一种效果
animation.type = @"rippleEffect";
animation.type = @"pageCurl";
animation.type = @"pageUnCurl"
animation.type = @"cameraIrisHollowOpen ";
animation.type = @"cameraIrisHollowClose "; |
下图是第一个cube立方体的效果:
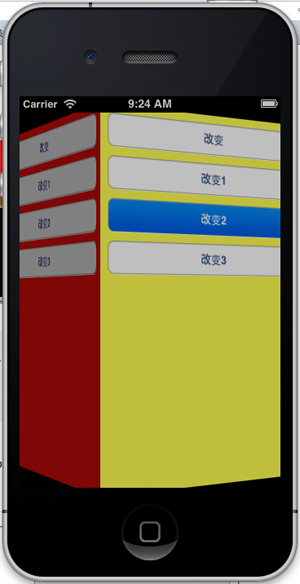
2.3 CATransition的 startProgress endProgress属性
这两个属性是float类型的。
可以控制动画进行的过程,可以让动画停留在某个动画点上,值在0.0到1.0之间。endProgress要大于等于startProgress。
比如上面的立方体转到,可以设置endProgress= 0.5,让动画停留在转动一般的位置。
上面这些私有的动画效果,在实际应用中要谨慎使用。因为在app store审核时可能会以为这些动画效果而拒绝通过。
3、UIView的 + (void)animateWithDuration
:(NSTimeInterval)duration animations:(void
(^)(void))animations completion:(void (^)(BOOL finished))completion方法。
这个方法是在iOS4.0之后才支持的。
比 1 里的UIView的方法简洁方便使用。
DidView里添加moveView。
moveView = [[UIView alloc] initWithFrame:CGRectMake(10, 180, 200, 40)];
moveView.backgroundColor = [UIColor blackColor];
[self.view addSubview:moveView]; |
- (void)changeUIView3{
[UIView animateWithDuration:3 animations:^(void){
moveView.frame = CGRectMake(10, 270, 200, 40);
}completion:^(BOOL finished){
UILabel *label = [[UILabel alloc] initWithFrame:CGRectMake(20, 20, 40, 40)];
label.backgroundColor = [UIColor blackColor];
[self.view addSubview:label];
}];
} |
然后用UIView animateWithDuration动画移动,移动动画完毕后添加一个Label。
3.2、 animateWithDuration的嵌套使用
- (void)changeUIView3{
[UIView animateWithDuration:2
delay:0
options:UIViewAnimationOptionCurveEaseOut animations:^(void){
moveView.alpha = 0.0;
}completion:^(BOOL finished){
[UIView animateWithDuration:1
delay:1.0
options:UIViewAnimationOptionAutoreverse | UIViewAnimationOptionRepeat
animations:^(void){
[UIView setAnimationRepeatCount:2.5];
moveView.alpha = 1.0;
}completion:^(BOOL finished){
}];
}];
} |
这个嵌套的效果是先把view变成透明,在从透明变成不透明,重复2.5次透明到不透明的效果。 |