ºÃ°É£¬¡°×î½ü¡±RESTºÜÈÈÃÅ...ÎÒÄÇôϲ»¶´ÕÈÈÄÖµÄÈË£¬µ±È»Ò²Ïë¸ãÒ»¸ã£¬Æäʵ×îÖ÷ÒªµÄÊÇSharePoint
2013ËÑË÷ÀïÃæÓÐÒ»ÖÖAPI²ÉÓÃÁËÕâÖÖÄ£ÐÍ£¬ÎªÁË¡°±¸×ÅÓá±£¬Ò²Ó¦¸ÃÁ˽âÏÂ...µ±È»Õâ¸öºÜ´ó³Ì¶ÈÉÏÊǸö½è¿Ú¡£°Ù¶ÈһϻòÕ߹ȸèһϽ̳ÌÕæ²»ÉÙ£¬È«²¿Éù³Æ¹¹½¨Ö§³Ö¡°CRUD¡±µÄREST
WCF£¬µ«ÊÇ´ò¿ªÒ»¿´£¬¼òÖ±¾ÍÊǿӵù°¡...ҪôֻʵÏÖÁ˲éѯ£¬ÒªÃ´Ö»ÊµÏÖÁË·þÎñûÓе÷ÓÃʾÀý£¬ÒªÃ´¾ÍÊÇžÓÃÒ»¸öÄ£°æÈ»ºó¸ÄÏ´úÂë...×îºóµ¼º½µ½·þÎñÒ³£¬Éù³Æ¹¹½¨Íê³É¡£µ±È»...¿´µ½¼¸Î»Ç°±²µÄÎÄÕ»¹ÊǺÜÈ«ÃæµÄ..Ö»²»¹ýÊDZȽϾɵķ½Ê½£¬½Úµã°ó¶¨±È½ÏÂé·³¾ÍûÓÐ×ßÄÇÌõ·¡£±¾ÎÄ¡°Ìص㡱£ºÕæÕýµÄCRUDÈ«°üº¬ÒÔ¼°µ÷Ó㬻õÕæ¼ÛʵͯÛÅÎÞÆÛ£»Ïêϸ¼Ç¼±¾È˵ÄÅÀ¿ÓÊ·£¨ÓÐЩʱºòÎÒÒ²²»ÖªµÀÎÒÔõôÅÀ³öÀ´µÄ£¬so...£©£»ÊÕ¼¸÷ÖÖ½â¾ö·½°¸µÄÁ´½Ó¡£
·þÎñ
Ä¿µÄÊÇÍê³ÉÒ»¸ö½Ð¡°BookService¡±µÄ·þÎñ£¬°üº¬»ù´¡µÄÔöɾ²é¸Ä²Ù×÷¡£Õû¸ö¿ÉÓõĽâ¾ö·½°¸Ö»ÐèÒª´¦Àí4¸öÎļþ£¬ËùÒÔûÓбØÒªÌṩÎļþÏÂÔØÖ»ÐèÒªÌù³öÔ´Âë¾ÍÐС£
[Êý¾Ý]ΪÁËÇø·ÖÖØµã£¬Ã»ÓбØÒªÊ¹ÓÃÊý¾Ý¿âÕâÑùµÄ¶«Î÷£¬Ê¹ÓôúÂëÒýÓÃÒ»¿éÄÚ´æµ±Êý¾Ý´æ´¢Çø¾Í¹»ÁË£¬ÏÈÊÇʵÌåÀ࣬´úÂëÈçÏ¡£
public class Book
{
static int id;
private Book(Book book)
{
//²ÎÊýֻΪǩÃû
}
public Book()
{
//Ψһ±ê¼Ç
id++;
Id = id;
}
public Book(string name, int saledCount)
:this()
{
//¹¹Ô캯Êý
Name = name;
SaledCount = saledCount;
}
public static Book Clone(Book book)
{
//¸´ÖƲ¿·ÖÊôÐÔ
Book clone = new Book(book);
clone.Id = book.Id;
clone.Name = book.Name;
return clone;
}
//Ö»ÓÐÄܹ»Get/SetµÄÊôÐÔ²ÅÄܱ»ÐòÁл¯
public int Id { get; set; }
public string Name { get; set; }
public int SaledCount { get; set; }
} |
»¹ÊÇ˵Ã÷Ï£¬static Book Clone·½·¨ÊÇΪÁËÌáÈ¡²¿·ÖÊôÐÔµÄ...ºóÀ´Á˽⵽¿ÉÒÔʹÓÃdynamic»òÕßResponseObject£»ÁíÍâÐèҪעÒâµÄÊÇ£¬¹«¿ªµÄ¾ßÓÐgetºÍset·ÃÎÊÆ÷µÄÊôÐÔ²ÅÄܳɹ¦±»ÐòÁл¯£¬±¾À´Ó¦¸Ãprivate
setµÄ£¬ÕâÀï¾ÍÖ»ÄÜget/setÁË¡£
[·þÎñ]¹ØÓÚÈçºÎ´ÓÒ»¸ö¿ÕµÄASP.NETÏîÄ¿¹¹ÔìÒ»¸öRESTful WCF·þÎñ£¬Çë²Î¿¼ÕâÀ·Ç³£Ïêϸ£ºhttp://geekswithblogs.net/michelotti/archive/2010/08/21/restful-wcf-services-with-no-svc-file-and-no-config.aspx¡£µ±È»ÕâÑùµÄ»°¾ÍÐèÒªÒýÓü¸¸ö³ÌÐò¼¯£º
System.ServiceModel
System.Web.Services
System.Web.Routing
System.ServiceModel.Activation
System.ServiceModel.Web
¿´ÍêÉÏÃæÄÇÆªÎÄÕ£¬¹¹½¨·þÎñÊÇûÎÊÌâµÄ£¬ÏñÎÒÕâôµô¿ÓµÄÈ˶¼Íê³ÉÁË¡£ÒÔÏÂÊÇÔ´Â룺
[ServiceContract]
public interface IBookService
{
[OperationContract]
Book GetBook(string id);
[OperationContract]
Book InsertBook(Book book);
[OperationContract]
void DeleteBook(string id);
[OperationContract]
IEnumerable GetAll();
[OperationContract]
void UpdateBook(Book book);
}
[ServiceBehavior(InstanceContextMode = InstanceContextMode.PerCall)]
[AspNetCompatibilityRequirements(RequirementsMode = AspNetCompatibilityRequirementsMode.Allowed)]
public class BookService : IBookService
{
#region ³õʼ»¯Ò»¿éÄÚ´æÊý¾Ý
static List BooksInMemory;
static BookService()
{
BooksInMemory = new List {
new Book("°ÍÀèʥĸԺ",10086),
new Book("СÍõ×Ó",2834),
new Book("¹ÊÊÂϸÄå",288347),
new Book("¼ÙÈç",1344),
};
}
#endregion
[WebGet(UriTemplate = "Book/{id}",
ResponseFormat = WebMessageFormat.Json,
RequestFormat = WebMessageFormat.Json)]
public Book GetBook(string id)
{
//R
var index = Int32.Parse(id);
return BooksInMemory.FirstOrDefault(i => i.Id == index);
}
[WebInvoke(UriTemplate = "Book", Method = "POST",
ResponseFormat = WebMessageFormat.Json,
BodyStyle = WebMessageBodyStyle.Bare,
RequestFormat = WebMessageFormat.Json)]
public Book InsertBook(Book book)
{
//C
var newBook = new Book()
{
Name = book.Name,
SaledCount = book.SaledCount
};
BooksInMemory.Add(newBook);
return newBook;
}
[WebInvoke(UriTemplate = "Book/{id}/", Method = "DELETE"
//ResponseFormat = WebMessageFormat.Json,
//BodyStyle = WebMessageBodyStyle.Bare
//RequestFormat = WebMessageFormat.Json
)]
public void DeleteBook(string id)
{
//D
int index = Int32.Parse(id);
var book = BooksInMemory.FirstOrDefault(i => i.Id == index);
if (book != null)
BooksInMemory.Remove(book);
}
[WebGet(UriTemplate = "Book/",
ResponseFormat = WebMessageFormat.Json,
RequestFormat = WebMessageFormat.Json)]
public IEnumerable GetAll()
{
//R
//¿ÉÒÔʹÓÃResponseObject£¬»òÕßdynamic
var set = BooksInMemory
//·µ»Ø²¿·ÖÊôÐÔ
.Select(i => Book.Clone(i));
return set;
}
[WebInvoke(UriTemplate = "Book", Method = "PUT")]
//BodyStyle= WebMessageBodyStyle.Bare,
//ResponseFormat = WebMessageFormat.Json,
//RequestFormat = WebMessageFormat.Json)]
public void UpdateBook(Book book)
{
//U
Book oldBook = BooksInMemory.FirstOrDefault(i => i.Id == book.Id);
if (oldBook != null)
{
oldBook.SaledCount = book.SaledCount;
oldBook.Name = book.Name;
}
}
} |
¿ÉÒÔ¿´µ½£¬ÎÒÔÚServiceµÄʵÏÖÀïÃæÉùÃ÷Á˾²Ì¬³ÉÔ±ÓÃÀ´´æ´¢Êý¾Ý¡£
[¿Ós]
POST£¬WebInvokeAttributeÓиö³ÉÔ±½ÐBodyStyle£¬ÏÈǰÎÒ¿´¹ýһƪÎÄÕÂ˵ÔÚXXÇé¿öÏÂÓ¦¸Ã½«BodyStyleÖÃΪWrapped£¬µ«ÊÇÎÒ·¸2ºöÂÔÁ˱ðÈË˵µÄÄǸöǰÖÃÌõ¼þ£¬ËùÒÔ±¯¾çÁË¡£ËùÒÔĿǰµÄÇé¿öÊÇ£¬½«BodyStyleÉèÖÃΪBare£¬Ò²¾ÍÊDz»°ü×°£¬È»ºóÔÚ¿Í»§¶ËʹÓò»°ü×°µÄjsonÇëÇóÌá½»¾Í¿ÉÒÔÁË¡£
PUT£¨DELETE£©£¬¾Í·þÎñÅäÖöøÑÔ£¬PUTÅÀ¿Ó»¨·ÑµÄʱ¼ä±È½ÏÉÙÁË...Ö÷ÒªÊÇÒªÅäÖÃIISÔÊÐíµÄ¶¯´Ê£¨verb£©£¬ÓÉÓÚÎÒʹÓõÄVS2012ËùÒÔµ÷ÊÔµÄʱºòʹÓÃÁËIIS
Express¡£¹ØÓÚÈçºÎÅäÖÃIIS ExpressµÄ¶¯´Ê¹ýÂ˹æÔò£¬²Î¿¼ÕâÀhttp://geekswithblogs.net/michelotti/archive/2011/05/28/resolve-404-in-iis-express-for-put-and-delete-verbs.aspx¡£ÁíÍ⣬²»ÐèÒªÉùÃ÷ÇëÇó¸ñʽºÍÏàÓ¦¸ñʽ£¨ºó»°£©¡£ÕâÀïÉÏÒ»ÕÅÅäÖÃconfigÎļþµÄ½ØÍ¼£º
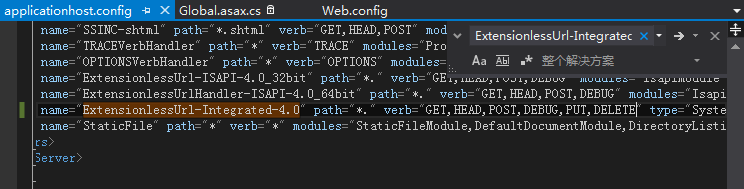
DELETE£¬ÖÁÓÚΪʲôҪ°ÑDELETEµ¥¶ÀÌá³öÀ´..ÄÇÊÇÒòΪÎÒ±»ÉîÉîµÄ¿ÓסÁË¡£ [WebInvoke(UriTemplate
= "Book/{id}/", Method = "Delete")]ÎÒʹÓÃÕâ¸öÌØÐÔµÄʱºò£¬Ò»Ö±·µ»Ø405
method not allowed£¬±È¶ÔϾÍÖªµÀÁË..´óСдÎÊÌâ°¡¡£ÎÒÒ²²»Çå³þÊÇʲôÇé¿öÁË..²»¹ýÎҲ²âÊÇIISÉÏÃæÅäÖõÄʱºòʹÓÃÁË´óдµÄdelete£¬ËùÒÔµ¼Ö±»¹ýÂËÁË¡£
ÁíÍ⣬¹ØÓÚPUTºÍDELETE£¬Ôø¾·µ»Ø¹ýÁ½¸ö´íÎó£¬Ò»¸öÊÇ404£¬ÁíÍâÒ»¸öÊÇ405¡£ÏÖÔÚÎÒÒѾ·Ö²»Çåʲôʱºò·µ»ØÊ²Ã´ÁË¡£ÍøÉÏÓÐЩ½â¾ö·½°¸ËµÒªÒƳýWebDAVºÍWebDAVMoudle£¬²Î¿¼ÕâÀhttp://forums.iis.net/post/1937843.aspx¡£ÆäʵÕâÁ½¸öÔÚIIS
ExpressÖÐÊÇÒѾ±»×¢Ê͵ôµÄ¡£ËùÒÔÖ÷Òª¹Ø×¢µãÔÚverbÅäÖÃÉÏ£¬ÎÒµÄweb.config¿ÉÒÔÖ¤Ã÷Õâµã¡£
<?xml version="1.0" encoding="utf-8"?>
<configuration>
<system.web>
<compilation debug="true" targetFramework="4.0"
/>
</system.web>
<system.webServer>
<modules runAllManagedModulesForAllRequests="true">
</modules>
</system.webServer>
<system.serviceModel>
<!--±ØÐëµÄ-->
<serviceHostingEnvironment aspNetCompatibilityEnabled="true"></serviceHostingEnvironment>
<standardEndpoints>
<webHttpEndpoint>
<!--1-->
<standardEndpoint name=""
crossDomainScriptAccessEnabled="true"
helpEnabled="true"
automaticFormatSelectionEnabled="true">
</standardEndpoint>
</webHttpEndpoint>
</standardEndpoints>
</system.serviceModel>
</configuration> |
[Global.asax]
Ö÷ÒªÊÇ´¦Àí·Óɵģº
using System;
using System.Collections.Generic;
using System.Linq;
using System.ServiceModel.Activation;
using System.Web;
using System.Web.Routing;
using System.Web.Security;
using System.Web.SessionState;
namespace MyRWfromEmt
{
public class Global : System.Web.HttpApplication
{
protected void Application_Start(object sender, EventArgs e)
{
RouteTable.Routes.Add(new ServiceRoute("", new WebServiceHostFactory(), typeof(BookService)));
}
#region
protected void Session_Start(object sender, EventArgs e)
{
}
protected void Application_BeginRequest(object sender, EventArgs e)
{
}
protected void Application_AuthenticateRequest(object sender, EventArgs e)
{
}
protected void Application_Error(object sender, EventArgs e)
{
}
protected void Session_End(object sender, EventArgs e)
{
}
protected void Application_End(object sender, EventArgs e)
{
}
#endregion
}
} |
µ÷ÓÃ
Õâ¸ö»¨Ñù¾Í¸ü¶àÁË£¬×÷Ϊһ¸ö½Å±¾²ËÄñ£¬²»ÏëÌÖÂÛÌ«¶à¡£ÕâÀïÉæ¼°Ò»ÏÂͬÓòµ÷ÓúͿçÓòµ÷ÓᣠÔÀíÇë²é¿´ÕâÀhttp://www.cnblogs.com/chopper/archive/2012/03/24/2403945.html¡£ÒòΪÊÇʹÓÃajax½øÐÐÇëÇó£¬Í¬Óòµ÷ÓþÍÈ·±£Ö´Ðнű¾µÄÒ³ÃæºÍ·þÎñ´¦ÓÚͬһ¸ö¶Ë¿Ú£¬¿çÓò¾ÍÊDz»Í¬¶Ë¿Ú£¨´ó¸ÅÊÇÕâôһ¸öÒâ˼°É£©¡£ÎÒÃÇÖ÷Òª´¦ÀíͬÓòµ÷Óá£
[ͬÓòµ÷ÓÃ]
ÏÈ¿´´úÂ룬Æäʵ¾ÍÊÇÒ»¸öhtmlÎļþ£¬È»ºóÖ´ÐÐÁËCRUDÕâЩ²Ù×÷£º
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0
Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title> RESTful WCF Service </title> <meta content="text/html; charset=utf-8" http-equiv="Content-Type" /> <script type="text/javascript" src="http://code.jquery.com/jquery-latest.js"></script> </head>
<body>
<div id ="actionarea">
</div>
<div id ="edit">
<input type="hidden" id="tid"
/>
ÊéÃû:<input name="Name" /><br
/>
ÊÛÁ¿:<input name="SaledCount" /><br
/>
<input type="button" onclick="sub();
return false;" value="Submit"/>
</div>
<input type="button" onclick="viewall();
return false;" value="ViewAll"/>
<input type ="button" onclick="ready4create();
return false;" value="Create" />
</body>
<!--½Å±¾-->
<script type="text/javascript">
$(function () {
$('#edit').hide();
});
//²é¿´È«²¿
function viewall()
{
$('#edit').hide();
$.getJSON('/book')
.done(function (data)
{
var html = "";
for (var i = 0 ; i < data.length; i++)
{
html += "<a href='#' onclick=\"viewsingle('"+data[i].Id+"');
return false;\">"
+ data[i].Name + "</a><br/>";
}
$('#actionarea').html(html);
});
}
//²é¿´µ¥Ìõ¼Ç¼
function viewsingle(id)
{
var uri = '/book/' + id;
$.getJSON(uri)
.done(function (data) {
var html = "Id:" + data.Id + "<br/>"
+ "Name:" + data.Name + "<br/>"
+ "SaledCount:" + data.SaledCount +
"<br/>";
html += "<a href='javascript:del("
+ data.Id + ");'>Delete</a>";
html += " ";
html += "<a href='javascript:ready4edit("
+ data.Id + ");' >Edit</a>";
$('#actionarea').html(html);
});
$('#edit').hide();
}
//ɾ³ý¼Ç¼
function del(id)
{
var uri = '/book/' + id;
$.ajax({
type: "Delete",
url: uri,
dataType: "text",
//processData:false,
contentType: "application/json;charset=utf-8",
success: function () {
viewall();
},
error: function (e) { document.write(e.ResponseText)
},
});
}
//Êä³öÏûÏ¢
function writemsg(data)
{
alert("e" + data);
}
//Ìá½»¼Ç¼
function sub() {
var mode = $('#tid').val() || "-1";
var name = $('input[name=Name]').val() || "";
var saledcount = $('input[name=SaledCount]').val()
|| "0";
var id = mode;
var message = "{\"Name\":\""
+ name + "\",
\"SaledCount\":"
+ saledcount + ",\"Id\":"
+ id + "}";
//¼òµ¥´¦Àí
if ((!mode) || mode == -1) {
//н¨
$.ajax({
type: "POST",
url: "/Book",
contentType: "application/json;charset=utf-8",
// processData: false,
data: message,
dataType: "json",
success:
function (data) {
$('input[name=SaledCount]').val('');
$('input[name=Name]').val('');
$('#edit').hide();
var html = "Id:" + data.Id + "<br/>"
+ "Name:" +
data.Name + "<br/>"
+ "SaledCount:" + data.SaledCount +
"<br/>";
html += "<a href='javascript:del("
+ data.Id + ");'>Delete</a>";
html += " ";
html += "<a href='javascript:ready4edit("
+ data.Id + ");' >Edit</a>";
$('#actionarea').html(html);
}
});
}
else {
//ÐÞ¸Ä
$.ajax({
type: "PUT",
url: "/Book",
contentType: "application/json;charset=utf-8",
processData: false,
cache:false,
data: message,
//Èç¹û½ö½öÖ¸¶¨jsonÔò¾ÍËãʼÖÕÒý·¢error
dataType: "text",
success: function () {
viewsingle(id);
},
error: function (e) { document.write(e.ResponseText)
},
});
}
}
//ÒªÇó±à¼
function ready4edit(id) {
$('#edit').show();
$('#actionarea').html('');
$('#tid').val(id);
$.getJSON("/Book/" + id)
.done(function (data) {
$('input[name=SaledCount]').val(data.SaledCount);
$('input[name=Name]').val(data.Name);
});
}
//ÒªÇó´´½¨
function ready4create() {
$('#edit').show();
$('#actionarea').html('');
$('#tid').val(-1);
}
$.ajaxSetup({
// Disable caching of AJAX responses
cache: false
});
</script>
</html> |
[¿Ós]
GET£¬ËµÀ´²ÑÀ¢£¬µ÷ÓÃGET·½·¨µÄʱºò¾ÍÒѾ´íÎ󯵳öÁË£¬Ö÷ÒªÊÇ£º1£¬ÂÒÂ룻2£¬ÌáʾȱÉٷֺţ¨;£©¡£ºóÀ´ÎÒûÓÐʹÓÃ$.ajax¶øÊÇʹÓÃÁË$.getJSON£¬´úÂë¶Ì²»Ëµ£¬»¹²»³ö´í¡£
POST£¬Ö÷ÒªÊÇdataµÄ¸ñʽ£¨²»°ü×°£©£¬Ó¦¸ÃÊÇ'{"XXX":int,"XX":"string"}'ÕâÑù£»ÓÐЩÎÄÕÂ˵Ӧ¸Ã½«processDataÖÃΪfalse£¬µ«ÊÇÎÒ·¢ÏÖ²»ÉùÃ÷ÕâÒ»ÌõÏÖÔÚÒ²¿ÉÒÔÕýÈ·Ö´ÐС£
PUT£¬Ç°ÃæËµ¹ý²»ÒªÖ¸Ã÷PUT·½·¨µÄResponseFormat£¬ÒòΪ·µ»ØµÄÊÇvoid¡£ËùÒÔÈç¹ûÔÚ¿Í»§¶Ëµ÷ÓõÄʱºòÖ¸Ã÷dataTypeΪ¡°json¡±£¬×ܻᴥ·¢errorʼþ£¬¾¡¹Ü·µ»ØµÄÊÇ200[OK]£¬Õâµã²Î¿¼ÕâÀ
http://stackoverflow.com/questions/6186770/ajax-request-return-200-ok-but-error-event-is-fired-instead-of-success£¬ÁíÍâÕâ¸ö±êÌâÖеÄfiredÕæÊÇ×ÅʵÈÃÎÒÀÖÁËһϣ¬ÀÏÍâÕæºÃÍæ£¨Ó¢ÓïºÃµÄÈË¿ÉÄܾõµÃÕâ¸ö´ÊºÜÕý³£°É£©¡£
DELETE£¬ºÍPUT²î²»¶à£¬Ö÷ÒªÊÇdataTypeµÄÎÊÌâ¡£
Ö´Ðнá¹ûÈçͼ£º
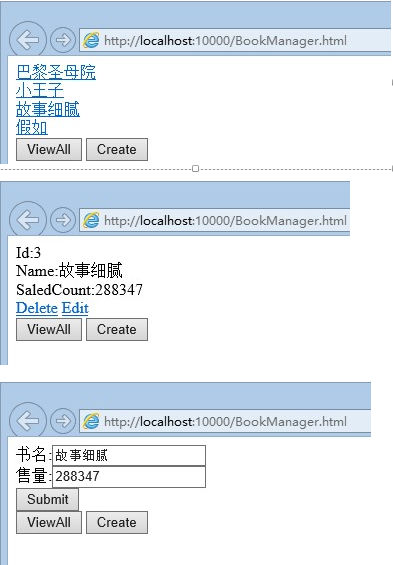
[¿çÓòµ÷ÓÃ]
ÕâÀïÔÙÒýÓÃһƪÎÄÕ£ºhttp://www.cnblogs.com/tom-zhu/archive/2012/10/25/2739202.html£¨ÅäÖã©¡£Ä¿Ç°ÎÒÃÇÊǰÑhtmlÎļþºÍservice·ÅÔÚͬһ¸ö½â¾ö·½°¸ÀïÃæ£¬È»ºóÔÚͬ¸ö¶Ë¿Ú´ò¿ª£¬ËùÒÔÊôÓÚͬÓòµ÷Óá£ÆäʵֻҪ°ÑhtmlÎļþÓÒ¼üʹÓÃä¯ÀÀÆ÷´ò¿ª¾ÍÊÇ¿çÓòµ÷ÓõÄÇéÐÎÁË¡£
ÖÁÓÚÔõôµ÷Óã¬Éϸö½ØÍ¼¾Í¿ÉÒÔһĿÁËÈ»£º
$.getJSONµ÷Óãº
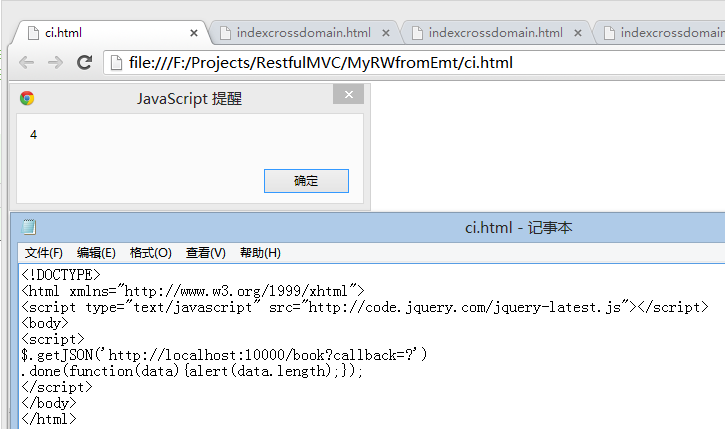
$.ajaxµ÷Óãº
$.ajax({
type:"GET",
url:'http://localhost:10000/book',
dataType:'jsonp',
success:function(e){alert(e.length)}
}) |
²¿Êð
[×ÔÍйܣ¨Self-Host£©]
ΪÁ˽â¾öÕâ¸öÎÊÌ⣬¼¸¸öСʱÓÖ¹ýÈ¥ÁË¡£µ«ÊÇ£¬·½·¨ÆäʵÊÇÒì³£¼òµ¥µÄ£¬ÇÒ΢ÈíµÄMSDNÉÏÓÐʾÀý£¬·Ç³£ÍêÕûµÄʾÀý¡£Çë²é¿´£ºhttp://msdn.microsoft.com/zh-cn/library/vstudio/ee662954(v=vs.100).aspx£¬ÕâÀïÊÇÒ»ÕûÌ׵ĴúÂë¡£ºÃÁË£¬ÕâÀïֻ˵×ÔÍйܡ£·½·¨ÊÇн¨Ò»¸ö¿ØÖÆÌ¨Ó¦ÓóÌÐò£¬È»ºóʹÓÃÒÔÏ´úÂ루Ìí¼Ó±ØÒªµÄÒýÓã©£º
using System; using System.Collections.Generic; using System.Linq; using System.ServiceModel; using System.ServiceModel.Description; using System.ServiceModel.Web; using System.Text;
namespace WCFConsole
{
class Program
{
static void Main(string[] args)
{
string uri = "http://localhost:9047";
using (WebServiceHost host = new WebServiceHost(typeof(MyRWfromEmt.BookService)
,new Uri(uri)))
{
host.Open();
Console.WriteLine("Please input exit to
close host.");
string key = Console.ReadLine();
while (key.ToLower() != "exit")
{
Console.WriteLine("Please input exit to close
host.");
key = Console.ReadLine();
}
}
}
}
} |
È»ºóÌí¼ÓÒ»¸öApp.configÎļþ£¬Ê¹ÓÃÈçÏÂÅäÖã¨ÆäʵºÍserviceµÄWeb.configʹÓõÄÅäÖÃÏàͬ<¸üÉÙ>£©£º
<?xml version="1.0" encoding="utf-8" ?> <configuration> <system.serviceModel> <standardEndpoints> <webHttpEndpoint> <!-- the "" standard endpoint is used by WebServiceHost for auto creating a web endpoint. --> <standardEndpoint name="" crossDomainScriptAccessEnabled="true" helpEnabled="true" automaticFormatSelectionEnabled="true"> </standardEndpoint> </webHttpEndpoint> </standardEndpoints> </system.serviceModel> </configuration> |
È»ºó¾Í¿ÉÒÔÖ´ÐÐÁË£¬Í¬Ñù£¬Ê¹ÓÃÒ»¸ö½ØÍ¼À´ËµÃ÷ÎÊÌ⣨ÕâÀïÊÇ¿çÓòµÄ£©£º
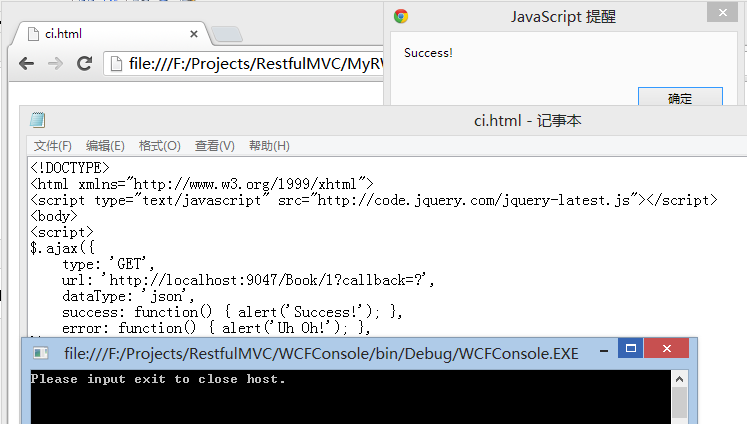
ÁíÍ⣬Èç¹ûûÓÐÔÚÅäÖÃÎļþÖÐÖ§³Ö¿çÓò£¬Æäʵ»¹ÊÇÄܹ»»ñµÃÊý¾Ý£¬Ö»ÊÇjavascript»á±¨Ò»¸ö´í£ºÈ±Éٷֺţ¨;£©£¬È»ºó×ÜÊÇ´¥·¢errorʼþ£¬ÓÚÊǾÍÎÞ·¨È¡µÃÖµÁË¡££¨ÕâÊÇʹÓÃ×ÔÍйܷ½Ê½³öÏÖµÄÇé¿ö£©¡£ÖÁÓÚΪʲôjsonpµ÷Óûá³öÏÖÎÊÌ⣬Çë²Î¿¼ÕâÆªÎÄÕ£ºhttp://stackoverflow.com/questions/7936610/json-uncaught-syntaxerror-unexpected-token?answertab=active#tab-top¡£
[IIS]
²¿Êðµ½IISÉÏÆäʵ»¹Í¦Ë³ÀûµÄ...ÒòΪµçÄÔÔø¾ÅäÖùýWCF£¬ËùÒÔһЩÎÊÌâ¾ÍûÓÐÔÙ³öÏÖÁË¡£ÐèҪעÒâµÄÊÇ£¬ÓÉÓÚÎÒÔÚservicesÕ¾µãÏÂÃæÐ½¨ÁËbookserviceÓ¦ÓóÌÐò£¬ËùÒÔÇëÇóURIÓ¦¸ÃÐÞ¸ÄΪ¡®/bookservice/book¡¯¡£
È»ºó¾ÍÐèÒªÅäÖö¯´Ê£¬µ«ÊÇÕâÀïÓÐÒ»¸öÎÊÌ⣬£¨win8µçÄÔ£©£¬Èç¹ûÓ¦ÓóÌÐò³Ø²»ÊǼ¯³Éģʽ£¬ÔòÕÒ²»µ½ÄǸöÅäÖÃÏÈç¹ûÊǼ¯³Éģʽ£¬ÓÖÌáʾ¡°ÎÞ·¨´ÓSystem.ServiceModel¡±ÖмÓÔØ¡°System.ServiceModel.
Activation.HttpModule¡±¡£Èç¹ûÊDz¿ÊðÔÚServer 2008Éϵ¹ÊÇÕý³££¬Ö»ÐèÒª²ÎÕÕIIS
ExpressÅäÖõÄÄÇÆªÎÄÕ¼´¿É¡£
|