Maze Diagram
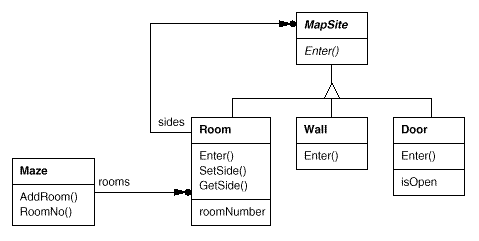
Maze.java
(without patterns)
Maze Diagram
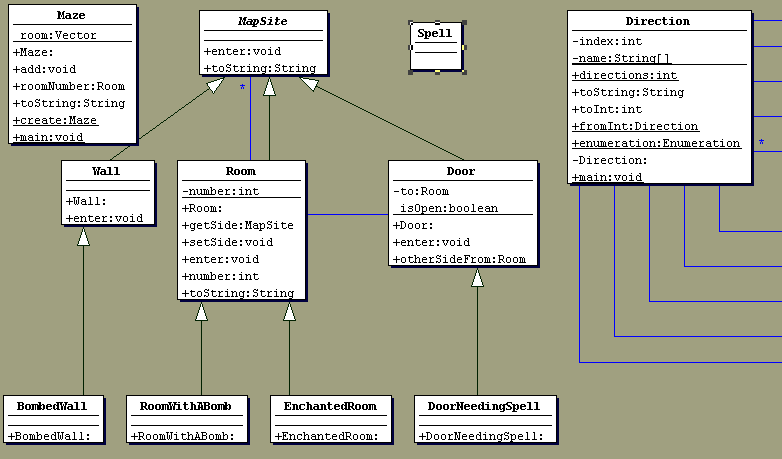
Analysis
The create() function is pretty complicated, considering that all
it does is create a maze with two rooms.
Inflexibility. It hard-codes the maze layout.
The creational patterns show how to make this design more flexible,
not necessarily smaller.
Suppose you wanted to reuse an existing maze layout for a new game
containing enchanted mazes.
How can you change CreateMaze easily so that it creates mazes with
these new classes of objects?
Use Creational Patterns
Creational patterns provide different ways to remove explicit references
to concrete classes from code that needs to instantiate them:
If CreateMaze calls virtual functions instead of constructor calls
to create the rooms, walls, and doors it requires, then you can
change the classes that get instantiated by making a subclass of
MazeGame and redefining those virtual functions. This approach is
an example of the Factory Method pattern.
If CreateMaze is passed an object as a parameter to use to create
rooms, walls, and doors, then you can change the classes of rooms,
walls, and doors by passing a different parameter. This is an example
of the Abstract Factory pattern.
If CreateMaze is passed an object that can create a new maze in
its entirety using operations for adding rooms, doors, and walls
to the maze it builds, then you can use inheritance to change parts
of the maze or the way the maze is built. This is an example of
the Builder pattern.
If CreateMaze is parameterized by various prototypical room, door,
and wall objects, which it then copies and adds to the maze, then
you can change the maze's composition by replacing these prototypical
objects with different ones. This is an example of the Prototype
pattern.