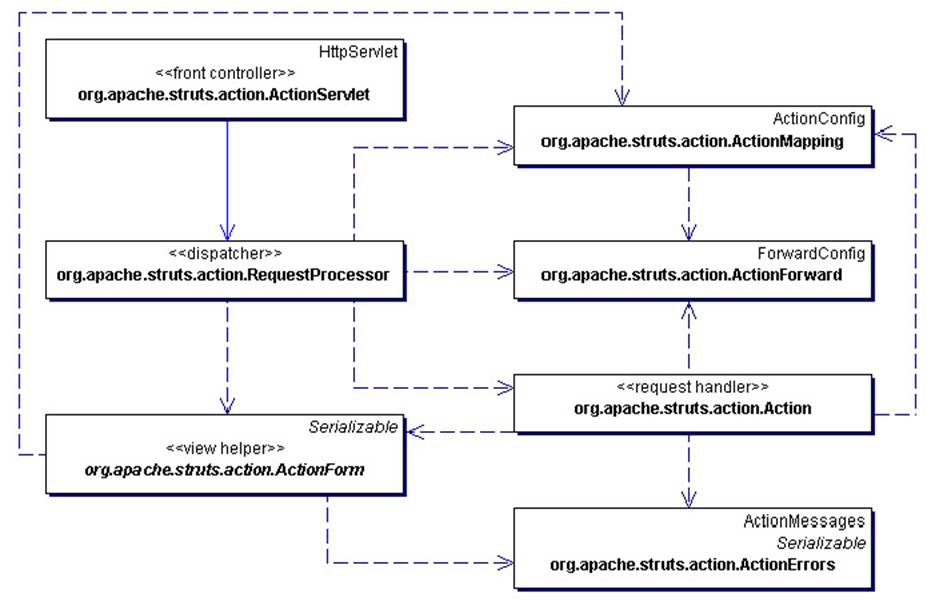
ActionServlet是一个FrontController,它是一个标准的Servlet,它将request转发给RequestProcessor来处理,
ActionMapping是ActionConfig的子类,实质上是对struts-config.xml的一个映射,从中可以取得所有的配置信息
RequestProcessor根据提交过来的url,如*.do,从ActionMapping 中得到相应的ActionForn和Action。然后将request的参数对应到ActionForm中,进行form验证。如果验证通过则调用Action的execute()方法来执行Action,最终返回ActionFoward。
ActionFoward是对mapping中一个foward的包装,对应于一个url
ActionForm使用了ViewHelper模式,是对HTML中form的一个封装。其中包含有validate方法,用于验证form数据的有效性。ActionForm是一个符合JavaBean规范的类,所有的属性都应满足get和set对应。对于一些复杂的系统,还可以采用DynaActionForm来构造动态的Form,即通过预制参数来生成Form。这样可以更灵活的扩展程序。
ActionErrors是对错误信息的包装,一旦在执行action或者form.validate中出现异常,即可产生一个ActionError并最终加入到ActionErrors。在Form验证的过程中,如果有Error发生,则会将页面重新导向至输入页,并提示错误。
Action是用于执行业务逻辑的RequsestHandler。每个Action都只建立一个instance。Action不是线程安全的,所以不应该在Action中访问特定资源。一般来说,应改使用 Business Delegate 模式来对Business tier进行访问以解除耦合。
Struts提供了多种Action供选择使用。普通的Action只能通过调用execute执行一项任务,而DispatchAction可以根据配置参数执行,而不是仅进入execute()函数,这样可以执行多种任务。如insert,update等。LookupDispatchAction可以根据提交表单按钮的名称来执行函数。
我们可以先回到刚才的例子,理解一下Struts的流程。
下面我们看Struts自带的example实例:
说明:实例二是Struts自带的example程序, 实现了登录,注册,修改功能。
代码中大量应用了struts taglib,并且采用validator插件进行form的验证。
但是代码树立了一个不好的榜样,即把大量的业务逻辑写在了action中。
部分代码如下:
登录:logon.jsp
<%@ page contentType="text/html;charset=UTF-8"
language="java" %> // 声明Taglib <%@ taglib uri="/WEB-INF/struts-bean.tld" prefix="bean" %> <%@ taglib uri="/WEB-INF/struts-html.tld" prefix="html" %> <html:html locale="true"> <head> // bean是用来从ApplicationResource中读取i18n信息 <title><bean:message key="logon.title"/></title> <html:base/> </head> <body bgcolor="white"> // 错误信息部分 <html:errors/> // 登录form,action为logion.do <html:form action="/logon" focus="username" onsubmit="return validateLogonForm(this);"> <table border="0" width="100%"> <tr> <th align="right"> <bean:message key="prompt.username"/>: </th> <td align="left"> <html:text property="username" size="16" maxlength="18"/> </td> </tr> <tr> <th align="right"> <bean:message key="prompt.password" bundle="alternate"/>: </th> <td align="left"> <html:password property="password" size="16" maxlength="18" redisplay="false"/> </td> </tr> <tr> <td align="right"> <html:submit value="Submit"/> </td> <td align="left"> <html:reset/> </td> </tr> </table> </html:form> // Validator插件,用于form验证 <html:javascript formName="logonForm" dynamicJavascript="true" staticJavascript="false"/> <script language="Javascript1.1" src="staticJavascript.jsp"></script> </body> </html:html> |
struts-config.xml配置
<form-beans> <!-- Logon form bean --> <form-bean name="logonForm" type="org.apache.struts.validator.DynaValidatorForm"> <form-property name="username" type="java.lang.String"/> <form-property name="password" type="java.lang.String"/> </form-bean> <!-- Subscription form bean --> <form-bean name="subscriptionForm"type="org.apache.struts.webapp.example.SubscriptionForm"/> </form-beans> <action-mappings> <!-- Edit mail subscription --> <action path="/editSubscription" type="org.apache.struts.webapp.example.EditSubscriptionAction" attribute="subscriptionForm" scope="request" validate="false"> <forward name="failure" path="/mainMenu.jsp"/> <forward name="success" path="/subscription.jsp"/> </action> ... |
subscriptionForm 是一个标准的ActionForm,其中reset方法用于清除form的值,validate方法用于验证
public final class SubscriptionForm extends
ActionForm { // The maintenance action we are performing (Create or Edit). private String action = "Create"; // Should we auto-connect at startup time? private boolean autoConnect = false; // The host name. private String host = null; private String password = null; private String type = null; private String username = null; public String getAction() { return (this.action); } public void setAction(String action) { this.action = action; } public boolean getAutoConnect() { return (this.autoConnect); } public void setAutoConnect(boolean autoConnect) { this.autoConnect = autoConnect; } public String getHost() { return (this.host); } public void setHost(String host) { this.host = host; } public String getPassword() { return (this.password); } public void setPassword(String password) { this.password = password; } public String getType() { return (this.type); } public void setType(String type) { this.type = type; } public String getUsername() { return (this.username); } public void setUsername(String username) { this.username = username; } /** * Reset all properties to their default values. * * @param mapping The mapping used to select this instance * @param request The servlet request we are processing */ public void reset(ActionMapping mapping, HttpServletRequest request) { this.action = "Create"; this.autoConnect = false; this.host = null; this.password = null; this.type = null; this.username = null; } /** * Validate the properties that have been set from this HTTP request, * and return an <code>ActionErrors</code> object that encapsulates any * validation errors that have been found. If no errors are found, return * <code>null</code> or an <code>ActionErrors</code> object with no * recorded error messages. * * @param mapping The mapping used to select this instance * @param request The servlet request we are processing */ public ActionErrors validate(ActionMapping mapping, HttpServletRequest request) { ActionErrors errors = new ActionErrors(); if ((host == null) || (host.length() < 1)) errors.add("host", new ActionError("error.host.required")); if ((username == null) || (username.length() < 1)) errors.add("username", new ActionError("error.username.required")); if ((password == null) || (password.length() < 1)) errors.add("password", new ActionError("error.password.required")); if ((type == null) || (type.length() < 1)) errors.add("type", new ActionError("error.type.required")); else if (!"imap".equals(type) && !"pop3".equals(type)) errors.add("type",new ActionError("error.type.invalid", type)); return (errors); } } |
logonAction
public final class LogonAction extends Action { /** * Process the specified HTTP request, and create the corresponding HTTP * response (or forward to another web component that will create it). * Return an <code>ActionForward</code> instance describing where and how * control should be forwarded, or <code>null</code> if the response has * already been completed. * * @param mapping The ActionMapping used to select this instance * @param form The optional ActionForm bean for this request (if any) * @param request The HTTP request we are processing * @param response The HTTP response we are creating * * @exception Exception if business logic throws an exception */ public ActionForward execute(ActionMapping mapping, ActionForm form, HttpServletRequest request, HttpServletResponse response) throws Exception { // Extract attributes we will need Locale locale = getLocale(request); MessageResources messages = getResources(request); User user = null; // Validate the request parameters specified by the user ActionErrors errors = new ActionErrors(); String username = (String) PropertyUtils.getSimpleProperty(form, "username"); String password = (String) PropertyUtils.getSimpleProperty(form, "password"); UserDatabase database = (UserDatabase) servlet.getServletContext().getAttribute(Constants.DATABASE_KEY); if (database == null) errors.add(ActionErrors.GLOBAL_ERROR, new ActionError("error.database.missing")); else { user = getUser(database, username); if ((user != null) && !user.getPassword().equals(password)) user = null; if (user == null) errors.add(ActionErrors.GLOBAL_ERROR, new ActionError("error.password.mismatch")); } // Report any errors we have discovered back to the original form if (!errors.isEmpty()) { saveErrors(request, errors); return (mapping.getInputForward()); } // Save our logged-in user in the session HttpSession session = request.getSession(); session.setAttribute(Constants.USER_KEY, user); if (log.isDebugEnabled()) { log.debug("LogonAction: User '" + user.getUsername() + "' logged on in session " + session.getId()); } // Remove the obsolete form bean if (mapping.getAttribute() != null) { if ("request".equals(mapping.getScope())) request.removeAttribute(mapping.getAttribute()); else session.removeAttribute(mapping.getAttribute()); } // Forward control to the specified success URI return (mapping.findForward("success")); } /** * Look up the user, throwing an exception to simulate business logic * rule exceptions. * * @param database Database in which to look up the user * @param username Username specified on the logon form * * @exception ModuleException if a business logic rule is violated */ public User getUser(UserDatabase database, String username) throws ModuleException { // Force an ArithmeticException which can be handled explicitly if ("arithmetic".equals(username)) { throw new ArithmeticException(); } // Force an application-specific exception which can be handled if ("expired".equals(username)) { throw new ExpiredPasswordException(username); } // Look up and return the specified user return ((User) database.findUser(username)); } } |